This Azure OpenAI and Blazor post will show how to create a Blazor web application and use Azure OpenAI.
About Blazor Application
The screenshot below displays a Blazor application that generates secure passwords by connecting to Azure OpenAI. The application requests a GPT-4 endpoint from the Azure OpenAI endpoint to create the password.
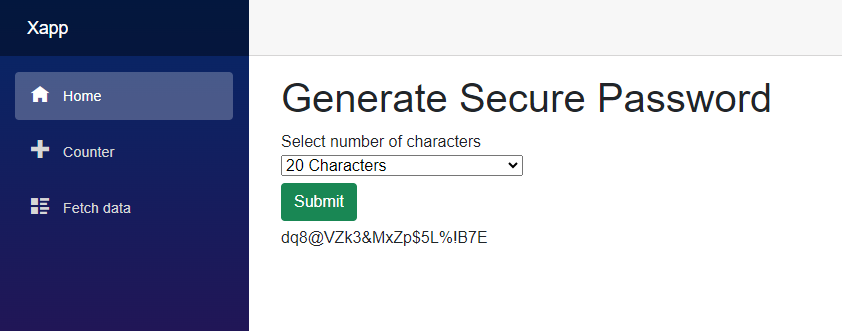
Components
The application has the following components
- Program.cs – Main C# file
- OpenAIService.cs – Class that connects and interacts with the Azure OpenAI endpoint.
- Index.razor – HTML and C# code the call the OpenAIService class
Use the above components. The application uses the Azure SDK for .NET to connect to an Azure OpenAI endpoint.
Note: The is using the Blazor WebAssambly template.
Prerequisites
Before you start, ensure you have an Azure OpenAI endpoint (use the post below if you don’t).
- Install the Azure OpenAI library
dotnet add package Azure.AI.OpenAI --version 1.0.0-beta.6
Create an appsettings.json under the wwwroot folder and store your Azure OpenAI endpoint and Key.
{
"OpenAIKey": "keygoeshere",
"OpenAIEndpoint": "https://url.api.cognitive.microsoft.com/"
}
Program.cs
using Microsoft.AspNetCore.Components.Web;
using Microsoft.AspNetCore.Components.WebAssembly.Hosting;
using Xapp;
using Xapp.Services;
var builder = WebAssemblyHostBuilder.CreateDefault(args);
builder.RootComponents.Add<App>("#app");
builder.RootComponents.Add<HeadOutlet>("head::after");
builder.Services.AddScoped(sp => new HttpClient { BaseAddress = new Uri(builder.HostEnvironment.BaseAddress) });
// Set up the OpenAI client
OpenAIService svc = new OpenAIService();
builder.Services.AddSingleton<OpenAIService>(svc);
var app = builder.Build();
var config = builder.Configuration;
var openAIKey = config["OpenAIKey"]!;
var openAIEndpoint = config["OpenAIEndpoint"];
svc.Initialize(openAIKey, openAIEndpoint);
await app.RunAsync();
Save the file and go ahead and create the class.
OpenAIService.cs
Note: In line 28, you need to enter the name of the LLM model you use; in our case, it’s gpt4.
using Azure.AI.OpenAI;
using Azure;
namespace Xapp.Services;
public class OpenAIService
{
OpenAIClient client;
static readonly char[] trimChars = new char[] { '\n', '?' };
public void Initialize(string openAIKey, string openAIEndpoint )
{
client = !string.IsNullOrWhiteSpace(openAIEndpoint)
? new OpenAIClient(
new Uri(openAIEndpoint),
new AzureKeyCredential(openAIKey))
: new OpenAIClient(openAIKey);
}
internal async Task<string> CallOpenAIChat(string passwordRequirements)
{
string prompt = GeneratePrompt(passwordRequirements);
ChatCompletionsOptions options = new ChatCompletionsOptions();
options.ChoiceCount = 1;
options.Messages.Add(new ChatMessage(ChatRole.User, prompt));
var response = await client.GetChatCompletionsAsync(
"gpt4", // Model name
options);
StringWriter sw = new StringWriter();
foreach (ChatChoice choice in response.Value.Choices)
{
var text = choice.Message.Content.TrimStart(trimChars);
sw.WriteLine(text);
}
var message = sw.ToString();
return message;
}
private static string GeneratePrompt(string passwordRequirements )
{
return $"Generate a secure password with {passwordRequirements} characters";
}
}
Save the file and open the Index.razor page or Home.razor.
Index.razor
@page "/"
@using Xapp.Services;
@using System.ComponentModel.DataAnnotations;
@inject OpenAIService openAIService;
<PageTitle>Index</PageTitle>
<h1>Generate Secure Password</h1>
<EditForm OnValidSubmit="OnSubmit" Model="@passwordModel">
<DataAnnotationsValidator />
<ValidationSummary />
<div>Select number of characters</div>
<select name="charactes" @bind="@passwordModel.passwordRequirements">
<option value="">----Please choose an option----</option>
<option value="16" >16 Characters</option>
<option value="20" selected>20 Characters</option>
<option value="30">30 Characters</option>
</select>
<div style="margin: 5px 0px 5px 0px">
<button type="submit" class="btn btn-success">
Submit
</button>
</div>
</EditForm>
<div>@responseText</div>
@code
{
PasswordModel passwordModel = new PasswordModel();
string responseText = "";
async void OnSubmit()
{
responseText = "Generating secure password, please wait.";
var message = await openAIService.CallOpenAIChat(passwordModel.passwordRequirements);
responseText = message;
this.StateHasChanged();
}
public class PasswordModel
{
[Required]
public string passwordRequirements { get; set; } = "20";
}
}
More AI articles
- Get Started With Azure Open AI .NET SDK
- Use Azure OpenAI with C# Application
- Deploy Azure OpenAI Resource With Terraform
- Connect to Azure OpenAI Using Postman
- Azure AI vs Azure Open AI
- Azure AI Studio vs Microsoft Copilot Studio
- Get Started With Azure AI Studio
- Create Prompt Flow for an LLM App in Azure AI Studio
- Detect Language using Azure AI Services
- Use GitHub Copilot to Write and Manage Code
- Install and use GitHub Copilot with Visual Studio
- Build Copilot With Azure AI Studio and Add Your Data
- Create Azure OpenAI Resource Using Azure CLI
- How to use the AzureOpenAI Client Class
- List All Azure AI LLM Model List With Azure CLI
- Use Azure OpenAI with Blazor Application