In this Microsoft Azure openAI post, we will create and connect a C# .NET application to Azure AI ChatGPT.
Microsoft Azure AI Services include the groundbreaking OpenAI ChatGPT engine and services, allowing Microsoft customers to use Azure security, scalability, and scale to deliver AI services.
As part of .NET integration with OpenAI, we can use C# and other .NET tools to connect the Azure OpenAI services and use services like Chat, Completions, DALL-E and more.
Before you start and follow this post, you must set up an Azure OpenAI resource and deploy a generative AI model. You will also need your endpoint address and key.
Create a C# Application
The first step in getting Azure OpenAI to work with a C# application is creating a new Visual Studio project.
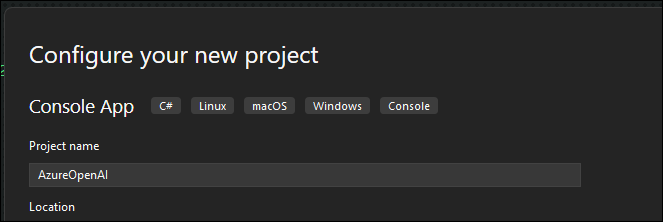
Install the OpenAI .NET Library
To connect to Azure OpenAI from a C# Application, we must install the .NET Azure OpenAI library using the following command or the NuGet Package Manager.
dotnet add package Azure.AI.OpenAI --prerelease
Once the package installation is completed, note your Azure OpenAI endpoint and key, as we will need them to create environment variables.
Create Environment Variables
Using the Azure OpenAI endpoint and key, use the following two PowerShell commands to create environment variables on your machine.
[System.Environment]::SetEnvironmentVariable('AZURE_OPENAI_KEY', 'REPLACE_WITH_YOUR_KEY_VALUE_HERE', 'User')
[System.Environment]::SetEnvironmentVariable('AZURE_OPENAI_ENDPOINT', 'REPLACE_WITH_YOUR_ENDPOINT_HERE', 'User')
.NET Code
The following C# code will use the OpenAI library and connect to the Azure OpenAI chat service. Make sure you replace the deployment name with your deployment.
using Azure;
using Azure.AI.OpenAI;
using static System.Environment;
string endpoint = GetEnvironmentVariable("AZURE_OPENAI_ENDPOINT");
string key = GetEnvironmentVariable("AZURE_OPENAI_KEY");
OpenAIClient client = new(new Uri(endpoint), new AzureKeyCredential(key));
var chatCompletionsOptions = new ChatCompletionsOptions()
{
Messages =
{
new ChatMessage(ChatRole.System, "How may I assist you today?."),
new ChatMessage(ChatRole.User, "Something here"),
new ChatMessage(ChatRole.Assistant, "Something here"),
new ChatMessage(ChatRole.User, "Something here"),
},
MaxTokens = 100
};
Response<ChatCompletions> response = client.GetChatCompletions(
deploymentOrModelName: "REPLACE_WITH_YOUR_DEPLOYMENT_NAME",
chatCompletionsOptions);
Console.WriteLine(response.Value.Choices[0].Message.Content);
Console.WriteLine();
Save the project and run it.
Leave a Reply