This Microsoft Azure AI Services and .NET blog post will show how to detect a language using the Azure AI Services SDK for .NET.
Azure AI Services offers a wide range of AI services, including the AI-Language Detection API, which can identify text input. The API can detect a language from a submitted text or a document with up to 5,120 characters.
This post will use the Azure AI Services SDK for .NET, making the API’s interaction smooth.
Console App
In the following console app, we are using the following components,
- Appsettings.json – To store the Azure AI multiservice endpoint details
- program.cs – To run the code
- DetectLanguage.csproj – To store the libraries and appsettings file details
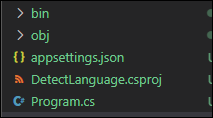
To get started, we need to create an Azure AI Multi Service resource to copy the endpoint and access key details.
Appsettings.json
Using the endpoint and access key details, create an appsettings.json file with the following configuration (replace with your endpoint and access key details).
{
"AIServicesEndpoint": "ENDPOINT URL",
"AIServicesKey": "ACCESS KEY"
}
DetectLanguage.csproj
Save the file, and copy the following code into yours .CSPROJ file.
<Project Sdk="Microsoft.NET.Sdk">
<PropertyGroup>
<OutputType>Exe</OutputType>
<TargetFramework>net8.0</TargetFramework>
<ImplicitUsings>enable</ImplicitUsings>
<Nullable>enable</Nullable>
</PropertyGroup>
<ItemGroup>
<PackageReference Include="Azure.AI.TextAnalytics" Version="5.3.0" />
<PackageReference Include="Microsoft.Extensions.Configuration.Json" Version="3.1.3" />
</ItemGroup>
<ItemGroup>
<None Update="appsettings.json">
<CopyToOutputDirectory>PreserveNewest</CopyToOutputDirectory>
</None>
</ItemGroup>
</Project>
Program.cs
Finally, use the following code in your program.cs file.
Note: The variable var text = “something” is the input we pass to the API to detect.
using System;
using System.IO;
using System.Text;
using Microsoft.Extensions.Configuration;
using Azure;
using Azure.AI.TextAnalytics;
namespace DetectLanguage
{
class Program
{
static void Main(string[] args)
{
try
{
// Get config settings from AppSettings
IConfigurationBuilder builder = new ConfigurationBuilder().AddJsonFile("appsettings.json");
IConfigurationRoot configuration = builder.Build();
string aiSvcEndpoint = configuration["AIServicesEndpoint"];
string aiSvcKey = configuration["AIServicesKey"];
// Create client using endpoint and key
AzureKeyCredential credentials = new AzureKeyCredential(aiSvcKey);
Uri endpoint = new Uri(aiSvcEndpoint);
TextAnalyticsClient aiClient = new TextAnalyticsClient(endpoint, credentials);
// Add the text variable here
var text = "I had the best day of my life. I wish you were there with me.";
// Detect language
DetectedLanguage detectedLanguage = aiClient.DetectLanguage(text);
Console.WriteLine($"\n Detected Language: {detectedLanguage.Name}");
}
catch (Exception ex)
{
Console.WriteLine(ex.Message);
}
}
}
}
Result
Save the files and run them with dotnet run
The output is shown below.
Detected Language: English