This article will show you how to create a basic ASP.NET 5 (ASP.NET Core 1.X) Form using Tag Helpers. We will form HTML elements based on a C# model and use standard Razor syntax in the markup. There are various options available when creating an ASP.NET 5 page, including [and not limited to] using [plain old] HTML, (tagged-based) Razor syntax, and a combination of both.
Tag Helpers
ASP.NET 5 Tag Helpers allow developers to create forms using server-side code and not rely on HTML. The form configuration is driven from a C# object to reduce the code needed to develop forms.
Tag Helpers using elements like <input> and <label> to link C# objects and forms, as you will see in the following example.
I have Tag Helpers example in the following example, which presents the user with a simple form that requires the user to provide their email address.
If required, the form details (Display name and validation is configured in the C# object (Input).
End Result
Before getting into the code, The screenshot below shows what we are going to achieve.
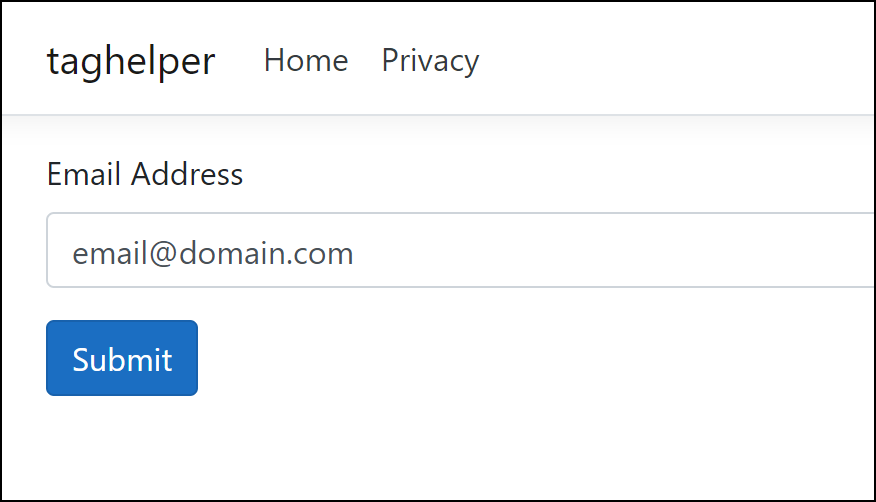
Code
The first piece of code I am going to share with you is the Razor view page.
In this code example, I’m creating the form on the Index page.
Index.cshtml
@page
@model IndexModel
@{
ViewData["Title"] = "Home page";
}
<form method="post" asp-antiforgery="true">
<div class="form-group ">
<label asp-for="Input.Email"></label>
<input class="form-control" asp-for="Input.Email">
<span asp-validation-for="Input.Email"></span>
</div>
<button type="submit" class="btn btn-primary">Submit</button>
</form>
The next code belongs to the C# Code-Behind page (Index.cshtml.cs)
The following namespace is needed for Data Annotations [EmailAddress] System.ComponentModel.DataAnnotations
using Microsoft.AspNetCore.Mvc;
using Microsoft.AspNetCore.Mvc.RazorPages;
using Microsoft.Extensions.Logging;
using System;
using System.Collections.Generic;
using System.ComponentModel.DataAnnotations;
using System.Linq;
using System.Threading.Tasks;
namespace taghelper.Pages
{
public class IndexModel : PageModel
{
[BindProperty]
public UserInputModel Input { get; set; } = new UserInputModel();
private readonly ILogger<IndexModel> _logger;
public IndexModel(ILogger<IndexModel> logger)
{
_logger = logger;
}
public void OnGet()
{
}
public class UserInputModel
{
[Required]
[EmailAddress]
[Display (Name = "Email Address") ]
public string Email { get; set; } = "email@domain.com";
}
}
}
At this stage, all you need to do is save the files and run the code. If you need to add more fields, follow the formula.