In this tutorial, we will show you how to create a simple form with ASP.NET Core 6 MVC.
ASP.NET Core is a powerful web development framework that makes it easy to create dynamic and responsive websites. Forms are an essential part of most websites, so learning how to create them using ASP.NET Core is a valuable skill. Let’s get started!
Creating a form in ASP.NET Core is simple. First, you need to create a new ASP.NET Core MVC project. You can do this using the Visual Studio IDE, dotnet CLI tool or PowerShell.
Once you have created your project, Create a class name formClass.cs and a Razor view called Form.cshtml
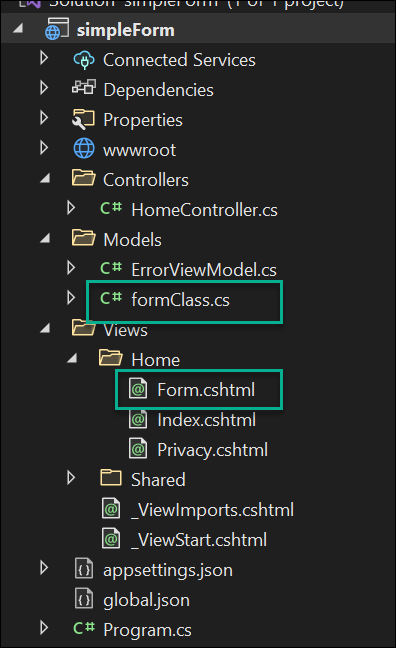
Code – formClass.cs
namespace simpleForm.Models
{
public class formClass
{
public string? Name { get; set; }
public string? Email { get; set; }
}
}
Code – Form.cshtml
@model simpleForm.Models.formClass
@{
Layout = null;
}
<!DOCTYPE html>
<html>
<head>
<style>
.myform{
border: 2px outset black;
background-color: white ;
text-align: center;
font-family: Arial;
}
.myforma {
font-size: large;
}
</style>
<meta name="viewport" content="width=device-width" />
<title>Form</title>
</head>
<body>
<div class="myform" >
<h1 class="display-4">Simple form</h1>
<form asp-action="form" method="post">
<div class="myforma">
<label asp-for="Name" class="" >Your name:</label>
<input asp-for="Name" />
</div>
<div class="myforma">
<label asp-for="Email">Your email:</label>
<input asp-for="Email" />
</div>
<div>
<p></p>
<button type="submit">Submit form</button>
<p></p>
</div>
</form>
</div>
</body>
</html>
Add the following code to HomeController.cs and Index.cshtml
HomeController.cs
using System.Diagnostics;
using Microsoft.AspNetCore.Mvc;
using simpleForm.Models;
namespace simpleForm.Controllers;
public class HomeController : Controller
{
private readonly ILogger<HomeController> _logger;
public HomeController(ILogger<HomeController> logger)
{
_logger = logger;
}
public IActionResult Index()
{
return View();
}
[HttpGet]
public ViewResult Form()
{
return View();
}
public IActionResult Privacy()
{
return View();
}
[ResponseCache(Duration = 0, Location = ResponseCacheLocation.None, NoStore = true)]
public IActionResult Error()
{
return View(new ErrorViewModel { RequestId = Activity.Current?.Id ?? HttpContext.TraceIdentifier });
}
}
Index.cshtml
@{
ViewData["Title"] = "Home Page";
}
<div class="text-center">
<h1 class="display-4">Welcome</h1>
<p>Learn about <a href="https://docs.microsoft.com/aspnet/core">building Web apps with ASP.NET Core</a>.</p>
<p> <a asp-action="form">Registration form</a> </p>
</div>
This code will create a simple form with two input fields: a text field, an email field plus a submit button. ASP.NET Core uses the Model-View-Controller (MVC) pattern.
The form will be submitted to itself.
To test your form, run the ASP.NET Core project and click on the Registration form link

The link will take you to the simple form as shown below.
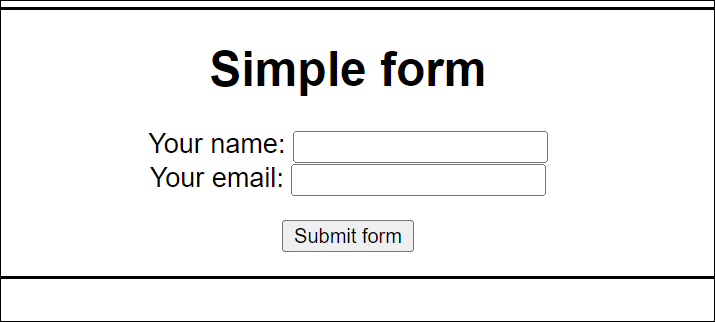
Congratulations! You have successfully created a simple form with ASP.NET Core MVC. In the next tutorial, we will show you how to validate form input using ASP.NET Core MVC. Stay tuned!